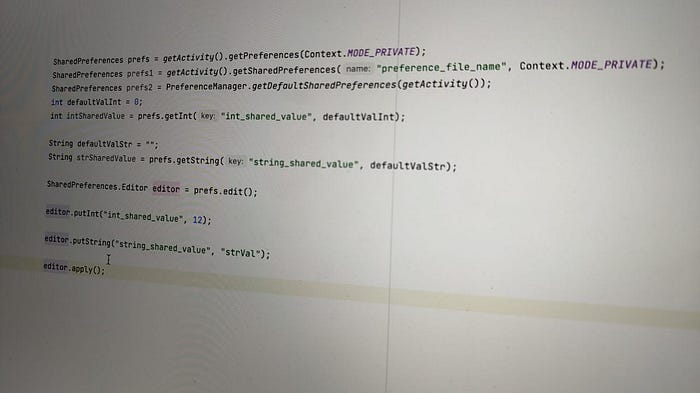
Introduction:
There are some instances where we have to store some key-value pairs in local database to access it very fast. Shared Preferences is one option where we can store key-value pair in local storage. It is a light weight database which is stored in the XML file in local storage.
How it works:
Shared preferences stores in key-value pair, that key-value pair stores in XML file. Now there are three different methods to access these files.
Activity Level File:
If you want to store some data at activity level then you can use getPreferences
method and use the shared preference to store/access data. Here one file in created for this particular activity and hence you don’t need to provide the file name in this method parameter.
Activity Level File:
If you want to store some data at activity level then you can use getPreferences
method and use the shared preference to store/access data. Here one file in created for this particular activity and hence you don’t need to provide the file name in this method parameter.
SharedPreferences prefs = getActivity().getPreferences(Context.MODE_PRIVATE);
Application Level:
There are some instances where we have to access data in different activities, at that time we have to place shared preference data outside the activity. Use this when you have to maintain data in multiple shared preference files.
getSharedPreferences
we can use this method and use shared preferences to store/access data using multiple shared preference files. In this method we have to pass the file name as a parameter.
SharedPreferences prefs = getActivity().getSharedPreferences(
getString(R.string.preference_file_name), Context.MODE_PRIVATE);
Application Level (Default):
This is also a application level shared preference level file. Default file is maintain at application level with some default shared preference file name.
SharedPreferences prefs = PreferenceManager.getDefaultSharedPreferences(context);
The difference between getSharedPreferences
and getDefaultSharedPreferences
is that we have to pass the filename parameter in getSharedPreferences
but not in getDefaultSharedPreferences
.
Read From Shared Preference file:
To retrieve data from shared preference files we have to access shared preference file first and then you have different methods to access different types of data, like getInt(), getString(), getBoolean(), etc.
SharedPreferences prefs = getActivity().getPreferences(Context.MODE_PRIVATE);
// --------- OR --------
// SharedPreferences prefs = getActivity().getSharedPreference("preference_file_name", Context.MODE_PRIVATE);
// --------- OR ---------
// SharedPreferences prefs = getActivity().getDefaultSharedPreferences(Context.MODE_PRIVATE);
int defaultVal = 0;
int intSharedValue = prefs.getInt(getString(R.string.int_shared_value), defaultVal);
String defaultVal = "";
String strSharedValue = prefs.getString(getString(R.string.string_shared_value), defaultVal);
Write to Shared Preference file:
To write some data into shared preference file we again have to access shared preference file first then have to create editor to edit into the file and then finally use the key-value methods to store into shared preference file like putInt(), putString, putBoolean().
SharedPreferences prefs = getActivity().getPreferences(Context.MODE_PRIVATE);
// --------- OR --------
// SharedPreferences prefs = getActivity().getSharedPreference("preference_file_name", Context.MODE_PRIVATE);
// --------- OR ---------
// SharedPreferences prefs = getActivity().getDefaultSharedPreferences(Context.MODE_PRIVATE);
SharedPreferences.Editor editor = prefs.edit();
editor.putInt(getString(R.string.int_shared_value), intVal);
editor.putString(getString(R.string.string_shared_value), strVal);
editor.apply();
//editor.commit(); we can also use this commit method to save our changes.
.commit() return boolean for success/failure.
.apply() does not return anything, you can just call and leave it.
Modes of accessing shared preferences:
There are different modes of accessing shared preferences:
- MODE_PRIVATE: If you want to access the shared preference only by calling application then you can use this mode. Using this mode you can maintain security.
- MODE_APPEND: This will append the new preference with the already existing preference.
- MODE_WORLD_READABLE: In this mode other application can read your shared preference values.
- MODE_WORLD_WRITEABLE: In this mode other applications can write into your shared preferences.
Data Persist:
Let us see how shared preference is maintaining data on different application conditions.
Shared preference is maintaining the data till the app uninstall or clear data. If you clear app’s cache then you will still have your shared preference data.
Conclusion:
You can use shared preference when you want to store small amount and light-weight data in key-value pair.
Hope you have good understanding of Shared Preference. 😊